Recorder View Controller
This guide shows you how to use our customizable View Controller to record multiple clips.
Features
- Record multiple video clips
- Record single video clip
- Stitch video recordings together into one single video file
- Toggle Flashlight
- Zoom
- Tap/Hold to record
- 3 and 10 seconds Timer
- Switch between different recording modes (defaults to 15/60 seconds)
- Autofocus / Tap to focus
- Import from camera roll
- Switch between front/back camera
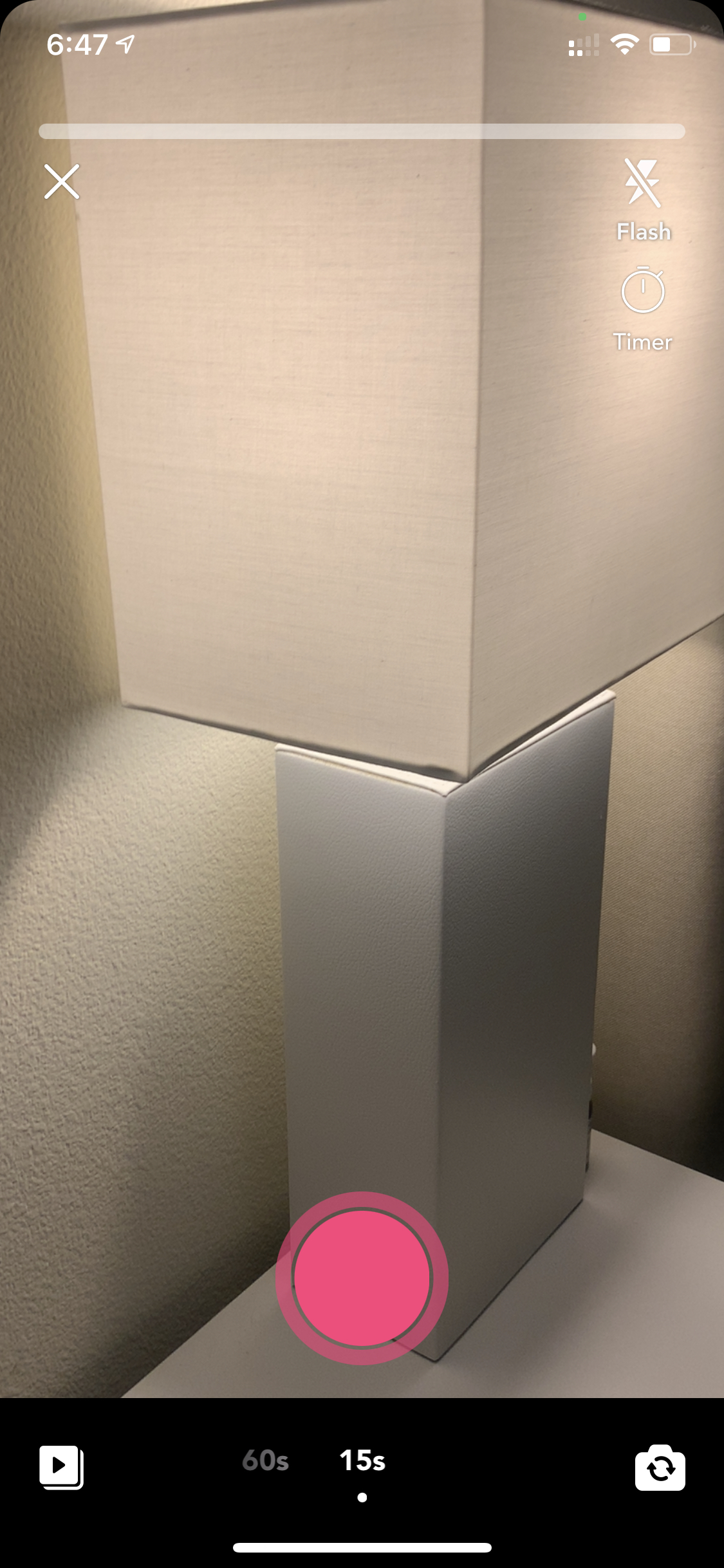
Usage
Create a new swift file RecorderViewController.swift with the following content:
RecorderViewController.swift
import UIKit
import VideoKitRecorder
class RecorderViewController: VKRecorderViewController, VKRecorderViewControllerDelegate, VKRecorderViewControllerDataSource {
/**
Set dataSource and delegate before super.viewDidLoad call.
*/
override func viewDidLoad() {
dataSource = self
delegate = self
super.viewDidLoad()
}
func shouldAutoMergeClips() -> Bool {
return true
}
func didFinishMergingClips(_ recorder: VKRecorder, mergedClipUrl: URL, autoMerged: Bool) {
print("Clips successfully merged into video file " + mergedClipUrl.absoluteString)
}
func didFailMergingClips(_ recorder: VKRecorder, error: Error, autoMerged: Bool) {
print("Failed merging clips.")
}
}
Your Recorder is now ready to be used.
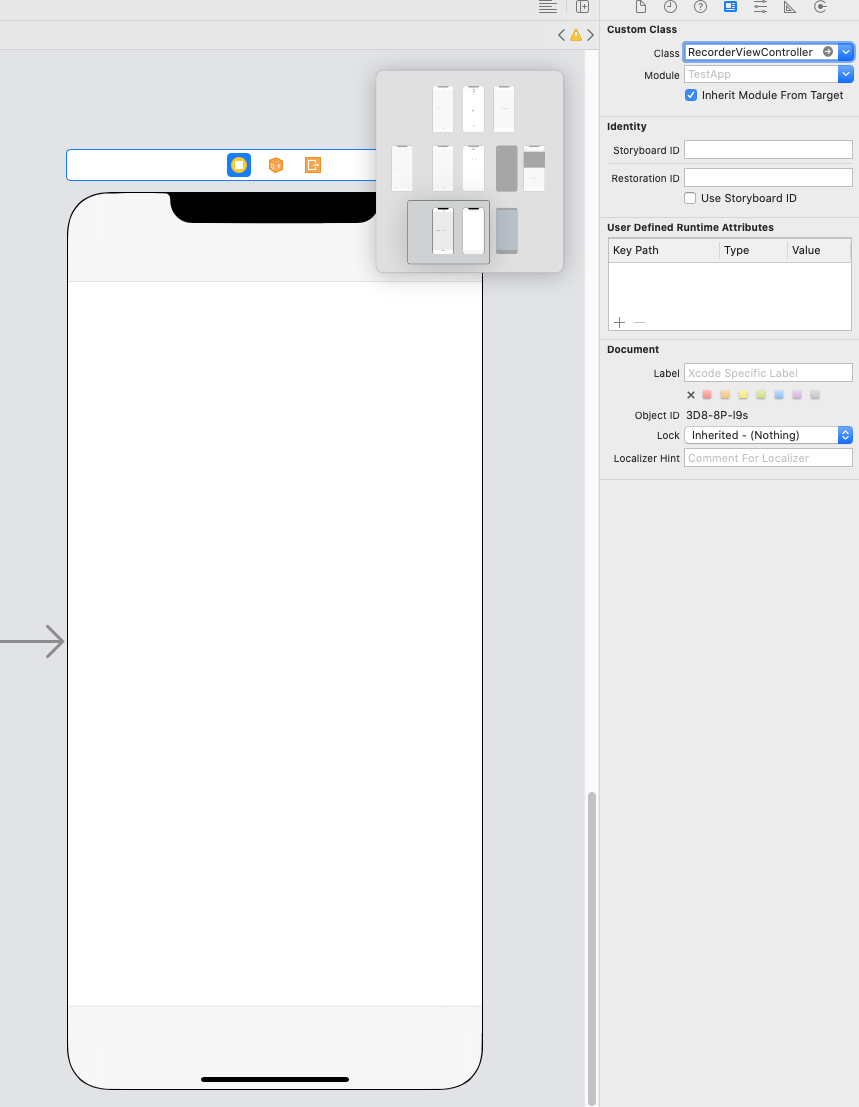
Customization
VKRecorderViewController
provides a variety of datasource methods so you can customize its look and feel and behaviour.
Just implement the following methods in accordance to the VKRecorderViewControllerDataSource
.
func shouldShowRecordButton() -> Bool {
return true
}
func shouldShowTimeSelector() -> Bool {
return true
}
func shouldShowFlashButton() -> Bool {
return true
}
func shouldShowTimerButton() -> Bool {
return true
}
func shouldShowPhotoLibraryButton() -> Bool {
return true
}
func shouldShowFlipCameraButton() -> Bool {
return true
}
func shouldAutoMergeClips() -> Bool {
return true
}
func exitMenuStartOverString() -> String {
return "Start New"
}
func exitMenuCancelString() -> String {
return "Abort"
}
func exitMenuExitString() -> String {
return "Close"
}
Delegates
Get full control over the recording by implementing any of the following VKRecorderViewControllerDelegate
delegate methods:
func didFinishMergingClips(_ recorder: VKRecorder, mergedClipUrl: URL, autoMerged: Bool) {
print("Clips successfully merged into video file " + mergedClipUrl.absoluteString)
}
func didFailMergingClips(_ recorder: VKRecorder, error: Error, autoMerged: Bool) {
print("Failed merging clips.")
}
func didSelectVideoFromCameraRoll(videoUrl: URL) {
print(#function)
}
func didSelectImageFromCameraRoll(image: UIImage) {
print(#function)
}
func didSelectRecordingLength(_ recordingLength: VKRecordingLength, picker: VKHorizontalPicker) {
print(#function + " - " + recordingLength.name)
}
func willStartCamera(_ recorder: VKRecorder) {
print(#function)
}
func didStartCamera(_ recorder: VKRecorder) {
print(#function)
}
func willStartRecording(_ recorder: VKRecorder) {
print(#function)
}
func didStartRecording(_ recorder: VKRecorder) {
print(#function)
}
func didPauseRecording(clip: VKRecorderClip, recorder: VKRecorder) {
print(#function)
}
func didSetupUI() {
print(#function)
}
func didSetupRecordButton(button: VKRecordButton) {
print(#function)
}
func configure(video: VKRecorderVideoConfiguration, audio: VKRecorderAudioConfiguration) {
print(#function)
}
func didTapNextButton(_ recorder: VKRecorder) {
print(#function)
}
func didExit(_ recorder: VKRecorder, recordingViewController: VKRecorderViewController) {
print(#function)
}
func exitMenuDidTapStartOver(_ recorder: VKRecorder) {
print(#function)
}
func exitMenuDidTapExit(_ recorder: VKRecorder) {
print(#function)
}